I spent most of my sixth week by writing shaders for blending part of tri planar texture mapping and completing my mid term goals. Clearing blending needs a lot of testing for a wide range of terrains but with my current pseudo random generator, terrain generation is restricted to some extent.
Initially the blending was done with the help of normals to the surface. The normal vector is normalized and broken into its components, and textures are applied in proportion to the blending values derived from normal vector components. Although technically correct, this blending technique was not so pleasant visually. Here is a code snippet for the process.
vec3 blending = getTriPlanarBlend(vNor.xyz );
vec4 xaxis = texture2D( colormap, vPos.yz );
vec4 yaxis = texture2D( colormap, vPos.xz );
vec4 zaxis = texture2D( colormap, vPos.xy );
vec4 colorTex = xaxis * blending.x + yaxis * blending.y + zaxis * blending.z;
From the getTriPlanarBlend function, we get the blend vector using the world space normal vectors. Then we take samples of the same texture in three different directions and we finally blend those textures to get the final texture. Here is a sample tile texture mapped on the terrain generated.
Here we can see a that most of the slopes are near 45 degrees and thus two or more textures are blended together to give a messed up look. A more focused blending is implemented where smaller components are eliminated and only larger components are considered. Here is another snippet to eliminate smaller vector components of the blending vector.
float threshold = 0.60;
if(blending.y > threshold){
blending.y = 1.0;
blending.x = 0.0;
blending.z = 0.0;
}
if(blending.z > threshold){
blending.z = 1.0;
blending.x = 0.0;
blending.y = 0.0;
}
if(blending.x > threshold){
blending.x = 1.0;
blending.y = 0.0;
blending.z = 0.0;
}
This is one of the blending corrections done to make the texture more usable.
But even this is not enough. This is just a fix for now but a more robust blending functionality is needed. We can make use of Texture Splatting but a lot of overhead processing and storage of an extra alpha channel might slow down the terrain generation. Though a look at the terrain after a few such corrections is better to get us moving.
But these not so curvaceous terrains are not good for any real purpose. A better looking marble noise can be used for more terrain like features. A sample screenshot is shown below(please ignore the texture stretching as I was using planar mapping because of broken code). Still these terrains can not look like a real life terrain because after all we are creating them out from a random set.
So most of the seventh week was spent playing and testing with different sine and cosine functions which utilize the current pseudo random generators. Here is one of the function used in generating marble noise. I have omitted the parameters for noise function.
Well I feel the need of developing a proper GUI for terrain generation and using interactive algorithms like marching cubes, but for now it is out of scope from my current project timeline. I will definitely try to implement some part of it after I complete my current milestones.
Initially the blending was done with the help of normals to the surface. The normal vector is normalized and broken into its components, and textures are applied in proportion to the blending values derived from normal vector components. Although technically correct, this blending technique was not so pleasant visually. Here is a code snippet for the process.
vec3 blending = getTriPlanarBlend(vNor.xyz );
vec4 xaxis = texture2D( colormap, vPos.yz );
vec4 yaxis = texture2D( colormap, vPos.xz );
vec4 zaxis = texture2D( colormap, vPos.xy );
vec4 colorTex = xaxis * blending.x + yaxis * blending.y + zaxis * blending.z;
From the getTriPlanarBlend function, we get the blend vector using the world space normal vectors. Then we take samples of the same texture in three different directions and we finally blend those textures to get the final texture. Here is a sample tile texture mapped on the terrain generated.
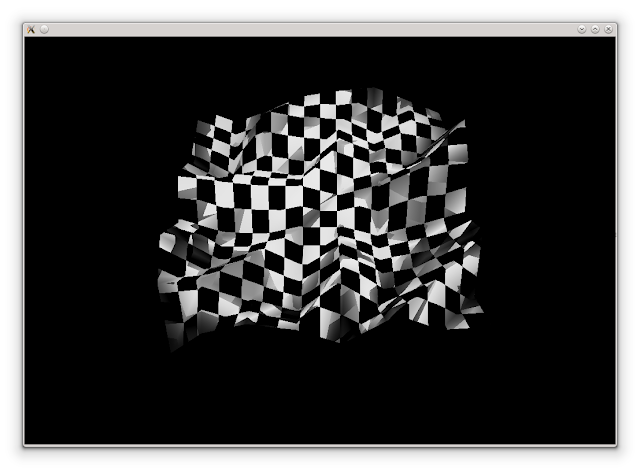
Here we can see a that most of the slopes are near 45 degrees and thus two or more textures are blended together to give a messed up look. A more focused blending is implemented where smaller components are eliminated and only larger components are considered. Here is another snippet to eliminate smaller vector components of the blending vector.
float threshold = 0.60;
if(blending.y > threshold){
blending.y = 1.0;
blending.x = 0.0;
blending.z = 0.0;
}
if(blending.z > threshold){
blending.z = 1.0;
blending.x = 0.0;
blending.y = 0.0;
}
if(blending.x > threshold){
blending.x = 1.0;
blending.y = 0.0;
blending.z = 0.0;
}
This is one of the blending corrections done to make the texture more usable.
But even this is not enough. This is just a fix for now but a more robust blending functionality is needed. We can make use of Texture Splatting but a lot of overhead processing and storage of an extra alpha channel might slow down the terrain generation. Though a look at the terrain after a few such corrections is better to get us moving.
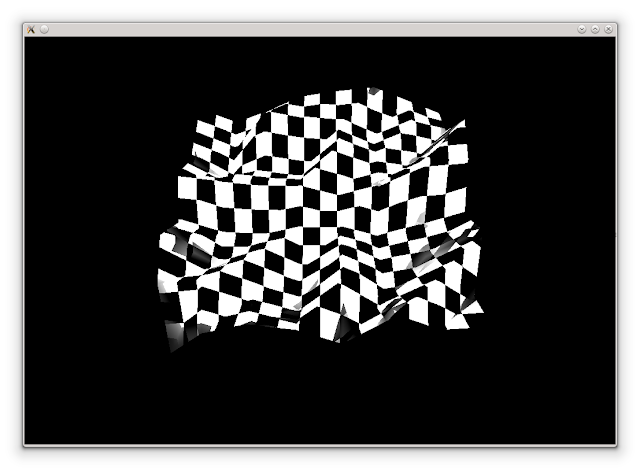
So most of the seventh week was spent playing and testing with different sine and cosine functions which utilize the current pseudo random generators. Here is one of the function used in generating marble noise. I have omitted the parameters for noise function.
Cos(x * scale/xdim + y * scale/ydim + twist * noise())
Well I feel the need of developing a proper GUI for terrain generation and using interactive algorithms like marching cubes, but for now it is out of scope from my current project timeline. I will definitely try to implement some part of it after I complete my current milestones.